Add a memo-function to store a number
Start my rearranging the buttons to make space for three new ones:
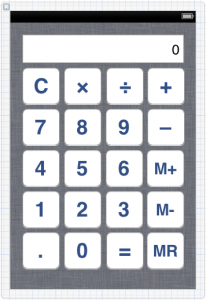
Create a variable to store the value:
float numberInMemory;
Initialize it:
- (void)viewDidLoad { ... numberInMemory = 0; }
And connect the buttons to suitable methods:
- (IBAction)addMemoryButtonPressed:(UIButton *)sender { numberInMemory += [self.numberTextField.text floatValue]; } - (IBAction)subtractMemoryButtonPresed:(UIButton *)sender { numberInMemory -= [self.numberTextField.text floatValue]; } - (IBAction)getMemoryButtonPressed:(UIButton *)sender { self.numberTextField.text = [NSString stringWithFormat:@"%@", [NSNumber numberWithFloat:numberInMemory]]; }













