Please note, this blog entry is from a previous course. You might want to check out the current one.
Your graphing view must be @IBDesignable and its scale must be @IBInspectable. The graphing view’s axes should appear in the storyboard at the inspected scale.
Add the respective keywords in the lines above the class and the property definitions:
@IBDesignable class GraphView: UIView { ... @IBInspectable var scale: CGFloat = 50.0 { didSet { setNeedsDisplay() } }
… and you should see the axis in storyboard, and when inspecting the graph view see the new property scale:
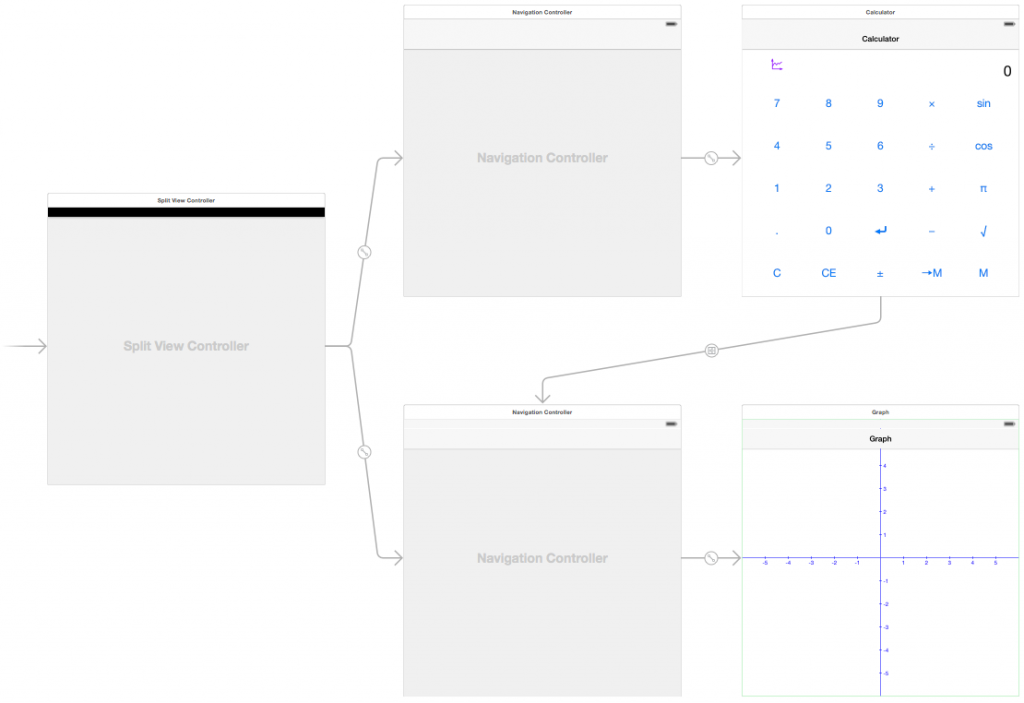
The complete code for the task #10 is available on GitHub.













