Please note, this blog entry is from a previous course. You might want to check out the current one.
Let the user choose how many cards to deal in the Playing Card game. Set is standardized to 12 cards to start a game, but the Playing Card game is flexible.
Because the specifications of the assignments call this tasks simple, choose the simplest implementation, using the game settings.
Add a new property to the game settings:
// GameSettings.h @property (nonatomic) int numberPlayingCards; // GameSettings.m #define NUMBERPLAYINGCARDS_KEY @"NumberPlayingCards_Key" - (int)numberPlayingCards { return [self getIntForKey:NUMBERPLAYINGCARDS_KEY withDefaultInt:22]; } - (void)setNumberPlayingCards:(int)numberPlayingCards { if (numberPlayingCards != self.numberPlayingCards) [self setInt:numberPlayingCards forKey:NUMBERPLAYINGCARDS_KEY]; }
In storyboard drag out a new label for the setting description, another one for its value and a slider:
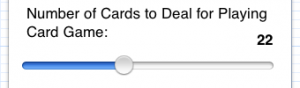
Create outlets to the value label and the slider:
@property (weak, nonatomic) IBOutlet UILabel *numberPlayingCardsLabel; @property (weak, nonatomic) IBOutlet UISlider *numberPlayingCardsSlider;
… as well as an action for when the value has changed:
- (IBAction)numberPlayingCardsChanged:(UISlider *)sender { [self setLabel:self.numberPlayingCardsLabel forSlider:sender]; self.gameSettings.numberPlayingCards = floor(sender.value); }
… and set them up when the setting views appears on screen:
- (void)viewWillAppear:(BOOL)animated { ... self.numberPlayingCardsSlider.value = self.gameSettings.numberPlayingCards; [self setLabel:self.numberPlayingCardsLabel forSlider:self.numberPlayingCardsSlider]; }
… and use the new setting in the playing-card-game view controller:
- (NSUInteger)startingCardCount { return self.gameSettings.numberPlayingCards; }
Note that you need to make gameSettings public in the super class!
The complete code is available on github.













